Whilst integrating Opayo (Formerly Sage Pay) using C# with .Net, I was having some issues with getting a payment registered using the "Form Integration" method, this included finding a definitive way to encrypt the "Crypt" field. With the help of the Opayo tech support, who are refreshingly responsive, who supplied an example encoded string, and looking at many code examples, we fixed all the issues.
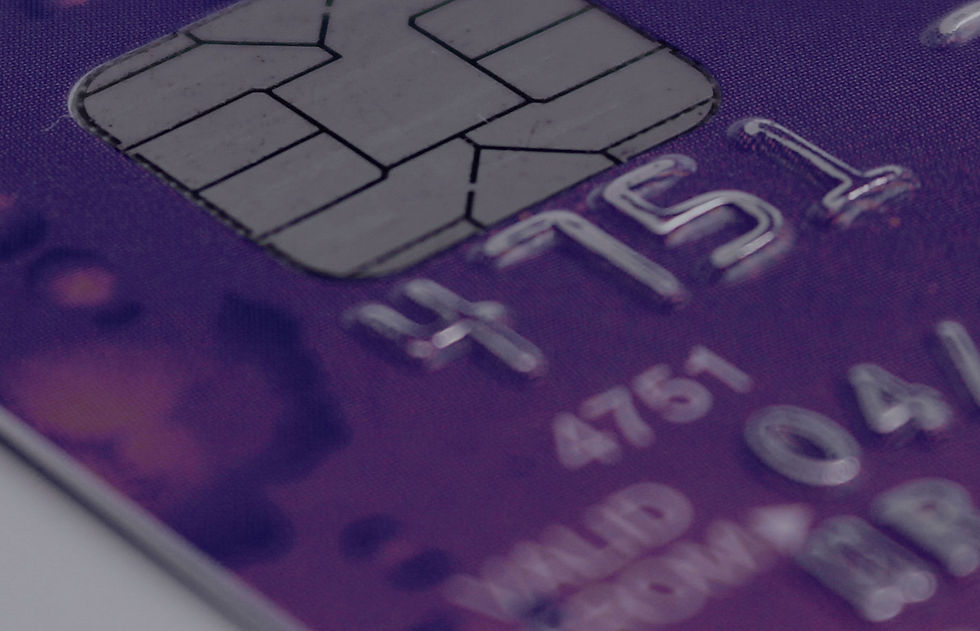
We are using the Opayo Form Integration, using the Version 4.00 protocol, Here are some things to check (and double check) 1) Ensure the correct Form value names are supplied when posting the form to register the payment. Form Name/Values VPSProtocol=4.00 (must be "4.00") TxType="PAYMENT" (for standard single payments) Vendor="YourVendorName" (the test account or live account vendor name) Crypt="@ENCRYPTED_NAME_VALUES"
2) The encryption algorithm. The encryption where ENCRYPTED_NAME_VALUES (above) are the required and optional name values for the transaction in a Url style string with Name=Value pairs separated by "&" encrypted using the code below.
The COFUsage field, indicated as non-mandatory in the Opayo online documentation does seem to be in fact a mandatory field in our current testing (TBC) we have set this to COFUsage=FIRST as we were getting the following error message in the admin console "3357 : The COFUsage field is missing"
Crypt Encryption Code
Below is a Crypt Class that will successfully encode/decode the crypt field. C# code below.. or latest on GitHub here: https://github.com/brightertools/OpayoCrypt/blob/main/Crypt.cs
using System;
using System.IO;
using System.Text;
using System.Security.Cryptography;
using System.Globalization;
namespace App.Opayo
{
public static class Crypt
{
public static string Encrypt(string input, string password)
{
return ByteArrayToHexString(AesEncrypt(Encoding.UTF8.GetBytes(input), password));
}
public static string Decrypt(string input, string password)
{
return Encoding.UTF8.GetString(AESdecrypt(ConvertHexStringToByteArray(input.Replace("@", "")), password));
}
private static byte[] ConvertHexStringToByteArray(string hexString)
{
if (hexString.Length % 2 != 0)
{
throw new ArgumentException("Binary key must have even number of digits");
}
byte[] data = new byte[hexString.Length / 2];
for (int index = 0; index < data.Length; index++)
{
string byteValue = hexString.Substring(index * 2, 2);
data[index] = byte.Parse(byteValue, NumberStyles.HexNumber, CultureInfo.InvariantCulture);
}
return data;
}
private static string ByteArrayToHexString(byte[] value)
{
return BitConverter.ToString(value).Replace("-", "");
}
private static byte[] AesEncrypt(byte[] input, string key)
{
using MemoryStream ms = new MemoryStream();
var aes = GetAesManagedEncryption(key);
CryptoStream cs = new CryptoStream(ms, aes.CreateEncryptor(), CryptoStreamMode.Write);
cs.Write(input, 0, input.Length);
cs.FlushFinalBlock();
return ms.ToArray();
}
private static byte[] AESdecrypt(byte[] input, string key)
{
using MemoryStream ms = new MemoryStream();
var aes = GetAesManagedEncryption(key);
CryptoStream cs = new CryptoStream(ms, aes.CreateDecryptor(), CryptoStreamMode.Write);
cs.Write(input, 0, input.Length);
cs.FlushFinalBlock();
return ms.ToArray();
}
private static AesManaged GetAesManagedEncryption(string key)
{
return new AesManaged
{
Padding = PaddingMode.PKCS7,
Mode = CipherMode.CBC,
KeySize = 128,
BlockSize = 128,
Key = Encoding.UTF8.GetBytes(key),
IV = Encoding.UTF8.GetBytes(key)
};
}
}
}
Hopefully this helps get your Opayo integration done faster.
תגובות